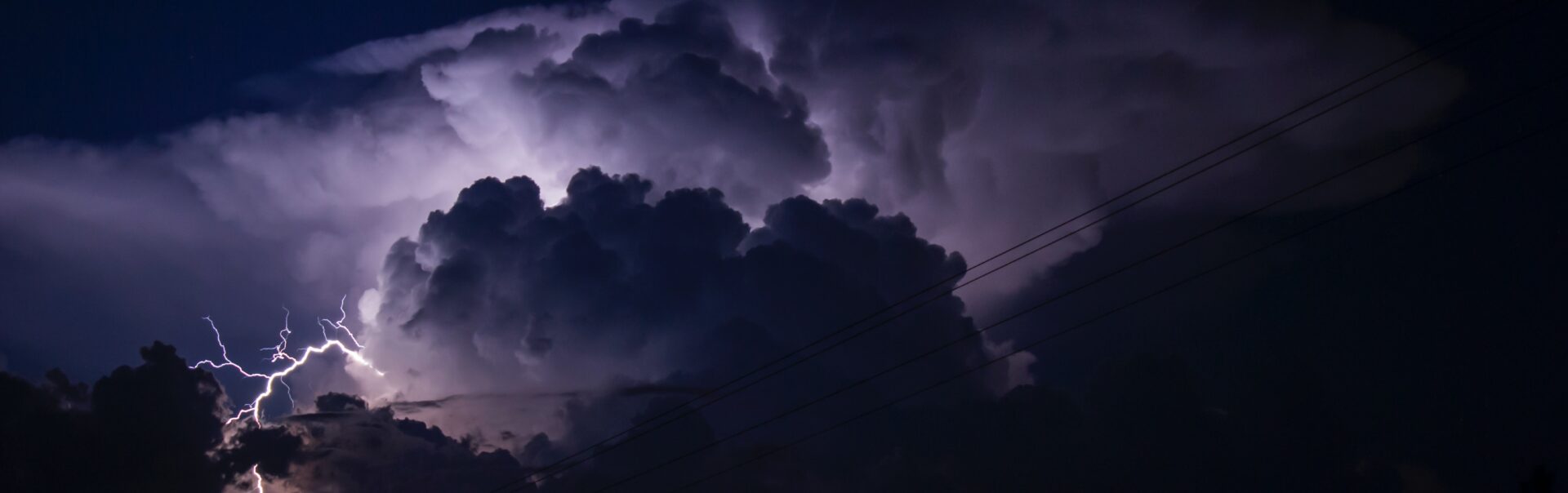
Articles
5 Steps to Make a Weather Data Component in React Apps
By eLearning Inside
September 23, 2021
Data services are an essential function of the web. People access the internet to discover a variety of information and insights, including seeking information such as weather.
On virtually all news sites, there is a component indicating the temperature and outside conditions in the city.
In this tutorial, we will present the 5 steps to build a component like this image.
Web components are functions that work in an encapsulated way. They have individual interoperability in comparison to other elements of the HTML page.
Some components can operate autonomously and customized with the native elements.
An API can operate custom components. The Application Programming Interface is a kind of messenger.
The main job is to make the connection between programs. The API requests to a data server the information, translates the data and returns it to the browser.
In 2021, the most common format is JavaScript Object Notation (JSON). JSON is an open file format that uses human-readable text. Data is transmitted in objectives that consist of attribute-value pairs and arrays.
The model has several advantages. A critical factor is how easy the API is to be integrated into the app. It was a fundamental step towards popularization.
1) Choosing One Cloud-Based API Service
The market trend is the emergence of dedicated API services. This type of job allows developers to rethink the role integration process.
Data-driven tools allow complete automation for companies.
An example of this type of service is apilayer, branded by Idera Devs Tools, which has a line of 20 types of API with several functions.
For weather data, one of the most popular options is Weatherstack.
According to apilayer, 75,000 companies use this API. The tool’s proposal is interesting: API meteorology data from around the world in real-time and historical.
The business can integrate the API with other tools such as PHP, Python, Nodejs, jQuery, Go, and Ruby.
2) Getting API authorization
First of all, the developer needs to make the register on the Weatherstack (https://weatherstack.com/signup/free).
In this tutorial, we will use the Free Plan. The service features paid monthly options that vary according to the number of API calls the application needs.
Some data is requested, such as email, name, and address.
The account login can access the API dashboard.
The dashboard allows querying the number of requests made from the API. The operation is intuitive.
The step to integration Weatherstack is to capture the API Access Key.
Access Key is the personal access key required by the tool for authentication.
The key is a string of 32 letters and numbers.
3) Setting the Endpoint
Now the developer needs to define the API endpoint. The endpoint is a URL link with the information to access the API data and some parameters about the necessary return.
It is the technology that allows the customization of requests.
In Weatherstack, the endpoint can choose between the current weather, historical weather, historical time-series, and weather forecast. Another parameter is the location lookup.
The primary request URL is http://api.weatherstack.com, which comes with the time parameter, a question mark, the access key, the ampersand, and the query.
The API URL endpoint format will be in the code as in the example below but without the spaces.
// Current Weather API Endpoint for New York
http://api.weatherstack.com/current
? access_key = 301faca57745d3aba9612fd6077d213f)
& query = New York
// optional parameters:
& units = m
& language = en
& callback = CALLBACK
Basically, what the developer need to do is get the URL (http://api.weatherstack.com/current?access_key =301faca57745d3aba9612fd6077d213f)& query=New York) and make a request in the application.
The dashboard allows querying the number of requests made from the API. The operation is intuitive.
4) Creating an Application Project
To start coding the component, we will use Create a New React App (https://reactjs.org/docs/create-a-new-react-app.html). The developer must have at least version 14 of Node and version 5.6 of npm installed on the machine.
When creating a project, it is possible to access a development environment with JavaScript resources.
A React application does not work with backend logic or a database. It creates a front-end pipeline to be used with implementing other functions.
npx create-react-app name_app
cd name_app
npm start
With these commands, the project file is created and runs in http://localhost:3000. The creation process may take a few minutes.
The coder can do project development in the preferred IDE (Integrated Development Environment). For this tutorial, we use Visual Code.
5) Coding the weather component
In the src folder, create a file .js named Weatherstack to code the component.
For this tutorial, we are going to use the native React styling. The developer can freely make the component with the look they want.
The first code to be created is a class component. In React, the coder can reuse components in other projects. It works like JavaScript but in isolation, with the HTML returns via the render() function.
import ‘./App.css’;
import React, { Component } from “react”;
class Weatherstack extends Component {
render() {
return <h2>Hi</h2>;
}
}
export default Weatherstack;
Next, we will define the constructor(), a method used to initialize the object’s state in a given class. In any constructor, the code must call super(props) before any other instruction. This code snippet is before render().
In it, we define what information the API returns we will use. In this case, they are the city, temperature, time icon, and date.
constructor(props) {
super(props);
this.state = {
city: [],
temperature: [],
date: [],
icon: []
};
}
To call the Weatherstack API data, we use componentDidMount().
This method is invoked immediately after the component is assembled. React is an option to instantiate a request if the project needs data from a remote endpoint.
To have the API interface, fetch is used, which allows the manipulation and access of data. In it, we indicate that endpoint captured on the API website.
The software can update variables defined in the constructor() within the set.State. It is necessary to check the JSON format of the Weatherstack API. Other information is provided by the API but will not be used in this project.
We indicate the location of the information in the variables as in the example below.
componentDidMount() {
fetch(
“http://api.weatherstack.com/current?access_key=301faca57745d3aba9612fd6077d213f&query=New York”
)
.then((res) => res.json())
.then((data) => {
this.setState({
city: data.location.name,
temperature: data.current.temperature,
date: data.location.localtime,
icon: data.current.weather_icons
});
});
}
In this part, the component can access the API data if it is put to run.
What the component lacks is the front-end itself. Inside render(), the collected information is highlighted.
render() {
const { city, temperature, date, icon } = this.state;
return (
<header className=”App-header”>
<h2>{temperature}ºC is the temperature now</h2>
<h2>in the city of {city}</h2>
<p>{date}</p>
<img src={icon} alt=”weather_icons” />
</header>
);
}
}
The component is made. Now it is time to import it into App.js. Before, it is recommended to delete the elements in this file that comes in React projects.
import ‘./App.css’;
import Weatherstack from ‘./Weatherstack.js’;
function App() {
return (
<div className=”App”>
<header className=”App-header”>
<Weatherstack/>
</header>
</div>
);
}
export default App;
The component is ready to be used in any project. On localhost, the developer can check the final result.
The cleanliness with which the API delivers data to the browser gives endless possibilities.
A front-end development is possible to present this information in the application in a fully customized way.
With API like this, the developer can deliver robust projects without spending hours building functions like this.
Featured Image: NOAA, Unsplash.
[…] Read the full story by eLearningInside News […]